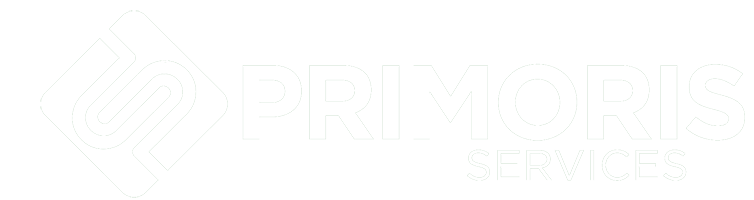
API Documentation
Accept Payments Anywhere & Anytime
Accept Payments Anywhere & Anytime
This document is for developers who wish to use the Primoris Payment API. If you are not a developer, but still wish to use the API, please contact us. We can help.
The Primoris Payment API processes single and batch credit card and electronic check payments, primarily for insurance policies. Payments are easily accepted from agents or individual insureds. The Payment API is simple and easy to use. You can get started in minutes and have a working system in almost no time.
To ensure data privacy, unencrypted HTTP is not supported. All requests, even in the testing environment, must be over HTTPS.
The Payment Card Industry Data Security Standard (PCI DSS) is a set of requirements designed to ensure that ALL companies that process, store or transmit credit card information maintain a secure environment. Primoris is PCI DSS complaint. Even if your company is not PCI DSS compliant, using the Primoris Payment API in accordance with this document will ensure compliance.
We designed the Primoris Payment API in a RESTful way, so that it is simple and straightforward to use. The API has 'endpoints', which are predictable, defined URLs to which requests can be made. JSON, a lightweight data format, will be returned from the API for all requests.
We assume you already have your API public/private key pair. If not, contact us and we will provide them for you.
Primoris issues API keys for each different configuration needed. For example, you might have multiple products that settle to different bank accounts. The API keys consist of a public key and a private key. The private key must be kept secret. No passwords are needed. You may have many active key pairs at any time.
Test transactions will not work until Primoris issues your keys.
The quickest way to get started is to use Primoris' PCI DSS compliant JavaScript library. By including this in your website, you can secure collection of payment information without concern for meeting PCI DSS compliance in your own facilities. The credit card or electronic check information that the user enters will never touch your servers - it will only pass through the secure, PCI DSS compliant, Primoris servers. This JavasScript embeds a payment form directly into your website, using the same technology that Google Maps uses to embed interactive maps into websites.
To see this in action, go to our demo site , select a sample policy, and sign in. On the second screen is the embedded payment form. Everything in gray is embedded and the data entered there will never touch your servers.
The script to embed is simply:
<script> PaymentPrefs = { embedWindow : "/paymentEmbed.php", actionPage : "/processPayment.php", // cancelPage link is optional. If you would like to hide this button, // simply comment this line out. cancelPage : "/cancelPayment.php", listeners : { // Binds a listener from an existing element (id) to an embed form element (key) // key: id // amount: 'paymentAmount' } } </script> <script src="https://frodo.dev.policy-service.com/assets/javascripts/controller.js" type="text/javascript" id="primoris_controller"></script> <div style="display:none;" id="loading"> Place any message or progress bar for payment processing here. The confirmation page expects an element with id="loading" </div>
Where the /paymentEmbed file, in PHP, is:
<!doctype html /> <html> <head></head> <body> <div id="paymentForm"> <script> PaymentPrefs = { pubkey : "$publicKey", // formID is only if you need the form variables // listed below to be injected into an existing form // formID: "paymentForm", view : "default", account_token : "$policy.accountToken", userCanSaveInfo : true, userCanPayWithPrepaid : true, readOnly : [ "amount" ], showAmount : true, showPhoneNumber : true, showEmail : true, // paymentType: // 1) is an optional field // 2) takes one of two params: "card", "bank" // 3) only works with the following views: "default", "recurring" paymentType: "card", amount : "$policy.formatted_amount", domain : "https://frodo.dev.policy-service.com", form : { name : "$policy.insuredName", address : "$policy.insuredAddress1", city : "$policy.insuredCity", state : "$policy.insuredState", zip : "$policy.insuredZip", account_number : "$policy.policyNumber", customer_identifier : "$policy.insuredID", user_identifier : "$user.userID", transaction_identifier : "$payment.paymentID" } } </script> <script src="https://frodo.dev.policy-service.com/assets/javascripts/embed.js" type="text/javascript" id="tolkien_embed"></script> </div> </body> </html>
The first section is setting up the associated files for processing the payment. The 'embedWindow' sets the path of the file for the embedded JavaScript. The 'actionPage' sets the path to the form action page in your site - there are form inputs that are created in the embed file that you can then use to POST form data in your site. Finally, there is the 'cancelPage' which is the path to a custom cancel page, if you have one. The cancel page may be left as an empty string if you don't have one.
The HTML form elements that are available to your action page are listed below. Note: the card number and the card security code are not available.
Primoris Payment API resources are identified as URLs that map to endpoints. You can access the resources using a web browser, curl, other command line tools, or through HTTP client tools, like Postman.
The requests endpoints are only available via the secure HTTPS protocol, and the domain names for the production and development endpoints, respectively, are:
paymentapi.policy-service.com // production frodo.dev.policy-service.com // development
All successful API responses will be in JSON format, wrapped in a "data" object if successful or an "error" object if not.
The account endpoint supports GET, POST, and PUT HTTP methods.
Returns a list of information for the specified {account}. The {account_token} is a alpha-numeric string 24 characters long.
attribute | type | description |
---|---|---|
key | string | The client's public key. [Required.] |
attribute | description |
---|---|
account_status |
The status of the account, either "active", "pending", or "revoked."
|
gateway_message | The human readable discription of what happened with the request. |
account_token | The account_token of the request. |
Creates a credit card or bank account_token.
attribute | type | description |
---|---|---|
key | string | The client's public key. [Required.] |
name | string | The full name of the card or bank account holder. 60 characters max. [Not required.] |
address | string | The card or bank account holder's billing address. This is used for Address Verification Service (AVS) on non-prepaid credit cards. 100 characters max. [Required for non-prepaid credit cards.] |
city | string | The card or bank account holder's billing city. 60 characters max. [Not required.] |
state | string | The card or bank account holder's billing state. 2 characters. [Not required.] |
zip | string | The card or bank account holder's billing zip code. 5 or 9 characters (no dash). [Required for non-prepaid credit cards.] |
string | The card or bank account holder's email address. This will be used to email a receipt. 200 characters max. [Not required.] | |
phone | string | The bank account holder's phone number. 25 characters max. [Not required.] |
amount | decimal | The amount to be charged, if making a sale. If included, this will also generate a payment_token, which can be used to process a single sale. [Not required.] |
installment_plan | string | The type of payment (one-time, recurring, or installment). [Required with amount field.] |
account_type | string | The type of the account, either "card" for credit card, "ACH" for debit payment or "bank" for bank account. [Required.] |
is_savings | boolean | Required for ACH, set yes or no. For 'account_type' bank, it is always false. |
card_number | digits | The credit card number. 16 characters max. [Required for credit card.] |
expiration_date | digits | The credit card's expiration date. 4 character string in MMYY format. [Required for credit cards.] |
cvv2 | digits | The credit card's security code. 3 or 4 digits. [Required for credit card.] |
account_name | string | The name of the bank for an electronic check. 200 characters max. [Required for e-check.] |
routing_number | digits | The bank routing number for electronic checks. 9 digits. [Required for e-checks.] |
bank_name | string | Entered by user. For e-check only. [Required for e-checks.] |
bank_account_number | digits | The bank account number for electronic checks 20 digits max. [Required for e-checks.] |
check_number | digits | The check number for electronic checks. This is only used if amount is also provided (i.e. a payment_token is generated too.) 6 digits max. [E-check only. Not required.] |
memo | string | The memo/text description for an electronic check. This is only used if amount is also provided (i.e. a payment_token is generated too.) 100 characters max. [E-check only. Not required.] |
account_number | string | The policy number/account number to which the payment applies. [Not required but strongly recommended for customer support.] |
customer_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
user_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
transaction_identifier | string | A system specific identifier for this specific transaction. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
prepaid | string | Prepaid cards are credit cards that are pre-loaded with money. The prepaid flag must be set to "yes" on any prepaid card transaction and "no" for any normal, non-prepaid card. [Required.] |
attribute | description |
---|---|
account_status |
The status of the account, either "active", "pending", or "revoked."
|
gateway_message | The human readable discription of what happened with the request. |
account_token | The newly created account_token, if approved. |
payment_token | The newly created payment_token. This is only provided if the amount is provided and the request is approved. |
Creates a payment_token for a single sale with an {account_token}.
attribute | type | description |
---|---|---|
key | string | The client's public key. [Required.] |
amount | decimal | The amount to be charged for a sale. [Required.] |
installment_plan | string | The type of payment (one-time, recurring, or installment). [Required with amount field.] |
check_number | digits | The check number for electronic checks. 6 digits max. [E-check only. Not required.] |
memo | string | The memo/text description for an electronic check. 100 characters max. [E-check only. Not required.] |
account_number | string | The policy number/account number to which the payment applies. [Not required but strongly recommended for customer support.] |
customer_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
user_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
transaction_identifier | string | A system specific identifier for this specific transaction. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
attribute | description |
---|---|
account_status |
The status of the account, either "active", "pending", or "revoked."
|
gateway_message | The human readable discription of what happened with the request. |
account_token | The account_token provided. |
payment_token | The newly created payment_token. |
Updates a credit card or bank account using the {account_token}.
attribute | type | description |
---|---|---|
key | string | The client's public key. [Required.] |
account_type | string | The type of the account, either "card" for credit card, "ACH" for debit payment, or "bank" for bank account. [Required.] |
is_savings | boolean | Required for ACH, set yes or no. For 'account_type' bank, it is always false. |
name | string | The full name of the card or bank account holder. 60 characters max. [Not required.] |
address | string | The card or bank account holder's billing address. This is used for Address Verification Service (AVS) on non-prepaid credit cards. 100 characters max. [Not required.] |
city | string | The card or bank account holder's billing city. 60 characters max. [Not required.] |
state | string | The card or bank account holder's billing state. 2 characters. [Not required.] |
zip | string | The card or bank account holder's billing zip code. 5 or 9 characters (no dash). [Not required.] |
string | The card or bank account holder's email address. This will be used to email a receipt. 200 characters max. [Not required.] | |
phone | string | The bank account holder's phone number. 25 characters max. [Not required.] |
status | string | This may be used to revoke an account, making it inactive. The value 'revoke" is the only valid input. Other values will be ignored. [Not required.] |
expiration_date | digits | The credit card's expiration date. 4 character string in MMYY format. [Required for credit cards.] |
account_name | string | The name of the bank for an electronic check. 200 characters max. [Not required.] |
routing_number | digits | The bank routing number for electronic checks. 9 digits. [Not required.] |
bank_account_number | digits | The bank account number for electronic checks 20 digits max. [Not required.] |
account_number | string | The policy number/account number to which the payment applies. [Not required but Strongly recommended for customer support.] |
customer_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
user_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
transaction_identifier | string | A system specific identifier for this specific transaction. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
attribute | description |
---|---|
account_status |
The status of the account, either "active", "pending", or "revoked."
|
gateway_message | The human readable discription of what happened with the request. |
account_token | The newly created account_token, if approved. |
The sale endpoint supports GET and POST methods.
Returns a list of information for the specified payment. The {payment_token} is a alpha-numeric string 24 characters long.
attribute | type | description |
---|---|---|
key | string | The client's public key. [Required.] |
attribute | description |
---|---|
source | The server environment that is used to process the sale. |
amount | The policy amount. This does not include the Primoris fee. |
primoris_fee | The Primoris fee, if charged to the insured, which is in addition to the policy amount. |
amount_total | The total amount charged, including the amount and the Primoris fee. |
authorization_code | The authorization code of the payment, if approved. This is an alpha-numeric string up to 10 characters. |
callID | A Primoris unique identifier for this specific transaction. The callID is used for every transaction type, not just payments. |
settlement_id | The unique settlement identifier for this payment, if the transaction has already settled. |
payment_detail_id | Another Primoris unique identifier for this specific payment. |
errors | An array of responses for this transaction. These responses are not necessarily failed transactions. |
payment_date | The date/time of the payment. |
payment_token | The payment_token entered on the request. |
payment_token_status |
The status of the payment_token. The values are either "active", "completed", or "revoked".
|
note | A note, if any. |
payment_name | The card or bank account holders name for this payment. |
confirmation_number | The confirmation number provided by the policy's system, indicating the payment has been applied to the policy. |
add_date | The date/time the record was added. |
installment_total | The total number of installments, if any. |
installment_made | The number of this specific installment, if any. |
installment_total | The total number of installments, if any. |
installment_plan | The name of the installment plan, if any. |
requests | The array of requests for this payment_token. |
requests -> transaction_identifier | The transaction_identifier for this request. |
requests -> account_number | The policy number or account number for this request. |
requests -> add_date | The date/time the record was added for this request. |
requests -> customer_identifier | The unique customer or insured identifier for this request. This is often the 'insuredID'. |
requests -> user_identifier | The unique user identifier for this request. This is the ID of the user logged into the client system who entered the payment. This is often the same as the customer_identified (if the insured is the one entering the payment.) |
Processes a sale for the specific {payment_token}.
Note: If a payment is processed multiple times, the Payment API
will return the original results of the request. The card or bank account will NOT be charged again.
attribute | type | description |
---|---|---|
private_key | string | The client's private key. [Required.] |
account_number | string | The policy number/account number to which the payment applies. [Not required but strongly recommended for customer support.] |
customer_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
user_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
transaction_identifier | string | A system specific identifier for this specific transaction. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
is_credit | boolean | Default is 'false'. All ACH transactions are debit unless this flag is set to ‘yes’. [Optional for ACH.] |
attribute | description |
---|---|
source | The server environment that is used to process the sale. |
amount | The policy amount. This does not include the Primoris fee. |
primoris_fee | The Primoris fee, if charged to the insured, which is in addition to the policy amount. |
amount_total | The total amount charged, including the amount and the Primoris fee. |
authorization_code | The authorization code of the payment, if approved. This is an alpha-numeric string up to 10 characters. |
callID | A Primoris unique identifier for this specific transaction. The callID is used for every transaction type, not just payments. |
settlement_id | The unique settlement identifier for this payment, if the transaction has already settled. |
payment_detail_id | Another Primoris unique identifier for this specific payment. |
errors | An array of responses for this transaction. These responses are not necessarily failed transactions. |
payment_date | The date/time of the payment. |
payment_token | The payment_token entered on the request. |
payment_token_status |
The status of the payment_token. The values are either "active", "completed", or "revoked".
|
note | A note, if any. |
payment_name | The card or bank account holders name for this payment. |
confirmation_number | The confirmation number provided by the policy's system, indicating the payment has been applied to the policy. |
add_date | The date/time the record was added. |
installment_total | The total number of installments, if any. |
installment_made | The number of this specific installment, if any. |
installment_total | The total number of installments, if any. |
installment_plan | The name of the installment plan, if any. |
requests | The array of requests for this payment_token. |
requests -> transaction_identifier | The transaction_identifier for this request. |
requests -> account_number | The policy number or account number for this request. |
requests -> add_date | The date/time the record was added for this request. |
requests -> customer_identifier | The unique customer or insured identifier for this request. This is often the 'insuredID'. |
requests -> user_identifier | The unique user identifier for this request. This is the ID of the user logged into the client system who entered the payment. This is often the same as the customer_identified (if the insured is the one entering the payment.) |
The confirmation endpoint supports the POST HTTP method. The confirmation process is used to verify that the successful payment has been applied to the policy/account.
Provides the Payment API with a confirmation number for a payment to indicate that the payment has been applied to the policy/account.
attribute | type | description |
---|---|---|
private_key | string | The client's private key. [Required.] |
payment_token | string | The payment_token for this confirmation_number. [Required.] |
confirmation_number | string | The confirmation number for this payment, indicating the payment has been applied to the policy/account. [Required.] |
account_number | string | The policy number/account number to which the payment applies. [Not required but strongly recommended for customer support.] |
customer_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
user_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
transaction_identifier | string | A system specific identifier for this specific transaction. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
attribute | description |
---|---|
data | A text response indicating the confirmation_number was applied to this payment. |
The refund endpoint supports the POST HTTP method. The refund will return a partial or full amount of the payment using the {payment_token}.
If a full refund is run before 12AM ET for a same-day sale transaction, the charge will not show up on the cardholder's statement. This is often referred to as a "void."
If the total amount charged or the policy amount is refunded, a full refund will be processed, including the refund of the Primoris fee.
Processes a partial or full refund on a complete payment using the {payment_token}.
attribute | type | description |
---|---|---|
private_key | string | The client's private key. [Required.] |
amount | decimal | The amount of the refund. Note: if eft blank, equals, or exceeds the amount of the payment, the full payment will be refunded. If this is less than the payment amount, a partial refund will be made. [Not required.] |
account_number | string | The policy number/account number to which the payment applies. [Not required but strongly recommended for customer support.] |
customer_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
user_identifier | string | A system specific identifier for this specific insured/customer. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
transaction_identifier | string | A system specific identifier for this specific transaction. This can help Customer Service identify payments. [Not required but strongly recommended for customer support.] |
attribute | description |
---|---|
source | The server environment that is used to process the sale. |
amount | The policy amount. This does not include the Primoris fee. |
primoris_fee | The Primoris fee, if charged to the insured, which is in addition to the policy amount. |
amount_total | The total amount charged, including the amount and the Primoris fee. |
authorization_code | The authorization code of the refund, if approved. This is an alpha-numeric string up to 10 characters. |
callID | A Primoris unique identifier for this specific transaction. The callID is used for every transaction type, not just payments. |
settlement_id | The unique settlement identifier for this payment, if the transaction has already settled. |
payment_detail_id | Another Primoris unique identifier for this specific payment. |
errors | An array of text responses for the refund. If approved, the text will be similar to, "Refund Request Accepted". |
payment_date | The date/time of the payment. |
payment_token | The payment_token entered on the request. |
payment_token_status |
The status of the payment_token. The values are either "active", "completed", or "revoked".
|
note | A note, if any. Successful refunds may have "refund" as the value. |
payment_name | The card or bank account holders name for this payment. |
confirmation_number | The confirmation number provided by the policy's system, indicating the refund has been applied to the policy. |
add_date | The date/time the record was added. |
installment_total | The total number of installments, if any. |
installment_made | The number of this specific installment, if any. |
installment_total | The total number of installments, if any. |
installment_plan | The name of the installment plan, if any. |
requests | The array of requests for this payment_token. |
requests -> transaction_identifier | The transaction_identifier for this request. |
requests -> account_number | The policy number or account number for this request. |
requests -> add_date | The date/time the record was added for this request. |
requests -> customer_identifier | The unique customer or insured identifier for this request. This is often the 'insuredID'. |
requests -> user_identifier | The unique user identifier for this request. This is the ID of the user logged into the client system who entered the payment. This is often the same as the customer_identified (if the insured is the one entering the payment.) |
The client endpoint supports the GET HTTP method.
Returns a list of information for the specific client and returns a list of fees for a specific payment amount for this client.
attribute | type | description |
---|---|---|
key | string | The client's public key. [Required.] |
amount | decimal | The policy payment amount for which all fees amounts will be returned. [Not required.] |
attribute | description |
---|---|
is_active | A yes/no indicator to show if this client record is active and permitted to process payments. |
customer_service_number | The Customer Service phone number for the client. |
is_active | A yes/no indicator to show if this client record is active and permitted to process payments. |
is_service_down | A yes/no indicator that shows if the client's service is unavailable. This is often used during server maintenance windows. |
min_payment | The minimum payment amount accepted for this client. |
max_payment | The maximum payment amount accepted for this client. This is ususally set as low as possible to minimize liability on fraudulent transactions. |
display_name | The client name that displays to the payee. |
fees | An array of fees for this client. |
fees -> fee_type | The type of fee, 'di' for Discover, 'mc' for MasterCard, 'vi' for Visa, and 'ec' for electronic check. |
fees -> customer_pays_fee | A yes/no indicator to show whether the customer pays the fee or the client pays the fee. |
fees -> amount | The amount of the fee. |
fees -> start_date | The date that this fee takes effect. |
fees -> end_date | The last date that this fee applies. |
fees -> is_holdback | A yes/no indicator to show if the fee is held back. |
fees -> fee_percent | If applicable, the percent of the amount used to calculate the fee. |
fees -> total_fee | The total fee that applies for the amount, if amount is passed in. |
The batch view endpoint supports the GET and POST HTTP method.
Returns a list of Payment Views (Sale GET Request) given a batch_id.
attribute | type | description |
---|---|---|
key | string | The client's public key. [Required.] |
batch_id | digits | The batch identifier returned by the Payment API on batch sale requests. [Required.] |
attribute | description |
---|---|
batch_id | The batch identifier returned by the Payment API on batch sale requests. |
batch_status | The current status of the batch. When this is "processed", all payments have been completed. |
add_date | The date/time the record was added. |
payments | A list of Payment Views (Sale GET Request) |
messages | A list of messages the system generated and tied to this specific batch. Typically used when something unexpected happened. |
Processes sale requests (Sale POST) for all listed accounts.
attribute | type | description |
---|---|---|
private_key | string | The client's private key. [Required.] |
data | json | A json list of Payment Creation Data (Account POST with account_token). [Required.] |
attribute | description |
---|---|
data -> batch_id | The batch identifier returned by the Payment API on batch sale requests to be used later in getting the batch/payment(s) status. |
data -> gateway_message | A message provided by the Payment API verifing the status of the batch |
Card Type | Card Number | CVV/CVC | Street Address | Zip Code |
---|---|---|---|---|
Visa | 4012301230123010 | 123 | 123 | 55555 |
Master Card | 5123012301230120 | 123 | 123 | 55555 |
American Express | 349999999999991 | 123 | 123 | 55555 |
Discover | 6011011231231235 | 123 | 123 | 55555 |
NOTE: It is important to start the street address with '123'. You can follow it with anything after.
For Example: 123 Main St.
The card associations have developed Address Verification as a tool to assist a merchant in processing a transaction based on information submitted that is compared to the credit card billing address. In order to test the variety of possible responses, the table below can be used. Defined input values will result in responses as described:
Street Address | Zip Code | AVS Result Code |
---|---|---|
123 | 55555 | Y – street and postal code match |
123 | 999991111 | Y – street and postal code match (Visa) X – street and postal code match (MasterCard) |
123 | EH8 9ST | D - exact match, international |
123 | Other Zip | A - address match, zip mismatch |
234 | Any Zip | U - address unavailable |
345 | Any Zip | G - verification unavailable due to international issuer non - participation (Visa and MasterCard only) |
456 | Any Zip | R - issuer system unavailable, retry |
235 | Any Zip | S – AVS not supported |
Other Address | 55555 | Z - address mismatch, 5-digit zip match |
Other Address | EH8 9ST | Z - address mismatch, international zip match |
Other Address | 999991111 | Z- address mismatch, zip match (Visa) W- address mismatch, zip match (MasterCard) |
Other Address | Other Zip | N - address and zip mismatch |
This table will test CVV, CVC, and CID which is the 3 or 4 digit code printed on the back of a card. This security feature assists merchants processing in a Card Not Present environment and receiving a positive response ensures the likely hood that the cardholder making the purchase is in physical possession of the card. Visa, MC, and Discover encode a 3 digit value on the cards and American Express encodes either a 3 or 4 digit value. Defined input values will result in responses as described:
CVV / CVC Value | Result Code |
---|---|
123 | M-Match |
234 | P-Not Processed |
345 | U-Issuer is not certified |
else | N-No Match |
This table will test American Express CID:
CID Value | Result Code |
---|---|
1234 | M-match (this response is generated when the CID flag is enabled on the MeS Profile ID) |
2345 | P-not processed (this response is generated when the CID flag is enabled on the MeS Profile ID) |
**** (any value other than 1234 or 2345) | N-no match (with a response code of 000) (this response is generated when the CID flag is enabled on the MeS Profile ID) |
This table will test CVV, CVC, and CID which is the 3 or 4 digit code printed on the back of a card. This security feature assists merchants processing in a Card Not Present environment and receiving a positive response ensures the likely hood that the cardholder making the purchase is in physical possession of the card. Visa, MC, and Discover encode a 3 digit value on the cards and American Express encodes either a 3 or 4 digit value. Defined input values will result in responses as described:
CVV / CVC Value | Result Code |
---|---|
123 | M-Match |
234 | P-Not Processed |
345 | U-Issuer is not certified |
else | N-No Match |
In addition to testing for approval codes, this table will allow testing for a wide variety of potential response codes:
Amount | Response Code | Auth response text | Reason/Description |
---|---|---|---|
0.00 | 085 | "Card OK" | For card verification testing, submit a transaction code=A |
0.01 | 001 | "Call" | refer to issuer |
0.02 | 002 | "Call" | refer to issuer - special condition |
0.04 | 004 | "Hold-call" | pick up card (no fraud) |
0.05 | 005 | "Decline" | do not honor |
0.07 | 007 | "Hold-call" | pick up card (fraud) |
0.14 | 014 | "Card No. Error" | Invalid card number |
0.15 | 015 | “No Such Issuer” | Invalid card number |
0.19 | 019 | "RE Enter" | re-enter transaction |
0.41 | 041 | "Hold-call" | pick up card (fraud: lost card) |
0.43 | 043 | "Hold-call" | pick up card (fraud: stolen card) |
0.51 | 051 | "Decline" | insufficient funds |
0.54 | 054 | "Expired Card" | issuer indicates card is expired |
0.57 | 057 | "Serv Not Allowed" | transaction not permitted to cardholder |
0.59 | 059 | “Suspected Fraud” | Do not honor card |
0.84 | 084 | “Invalid Auth” | Invalid Authorization Life Cycle |
0.86 | 086 | “Can’t Verify PIN” | System is unable to validate the Pin # |
1.10 | 0TA | "CT NOT ALLOWED" | Merchant does not accept this type of card |
The following AAV or ITD values will cause the simulator to return a 'Y' response in the appropriate response field.
Field Name | Group | Value |
---|---|---|
Billing Street Address | AAV | 123 (only numeric part matters) |
Billing Postal Code | AAV | 55555 or 999999999 |
Billing First Name | AAV | CHRIS |
Billing Last Name | AAV | FROST |
Billing Phone Number | AAV | 7035551212 |
Customer Email | ITD | cffrost@emailaddress.com |
Any other value will cause the simulator to return a 'N' response in the appropriate response field. If a given data element is not supplied the simulator will return a space.
The purpose of this section is to give some interactive feedback to the customers once they click the submit button. When the submit button is clicked, the data will be sent to the processor. This process will show some delay, during that time, it would be better if the customer looks at a 'loading' image.
Requirements For Loading Message
Here is an example of loading message code:
<div style="display: none;" id="loading"> Processing Payment<br /> <img alt="Loading" src="/images/busy.gif" width="220" height="19" /> <br /> </div>
Here is an example of loading message image:
The flow chart below indicates the repair process of point of sale payments with 'payment_status' 'active'.